Java中关于抛出异常的几个关键点
前言
编写代码过程中,使用事务回滚很常见,但关于事务回滚有几个很基础的问题
事务
错误示范
1 |
|
上述代码中,直接抛出了Exception
异常,这种事务是无法控制住的,无法进行回滚。
进入@Transactional
注解,其中有一个rollbackFor()
回滚,官方文档中规定了只会对运行时异常
和错误
进行回滚。
如果仅仅抛出一个Exception
受检异常,是无法进行回滚的。
正确示范
想实现事务回滚,必须要符合官方文档中规定的方式
例如:在注解中声明一下回滚异常类型,或抛出RuntimeException异常
方式一:注解规定类型
代码中虽然使用了Exception
异常,但是在注解中声明了方法中需要回滚的异常类型,所以下面的事务是可以回滚的
1 |
|
方式二:运行时异常
使用运行时异常时,直接默认符合官方文档中规定的事务回滚要求了
方法上也可以不需要声明 throws RuntimeException
继续向上抛异常了
1 |
|
方式三:自定义异常
CustomException
是自己定义的一个异常类,但自定义异常类一定要继承
运行时异常类(extends RuntimeException
)
所以自定义异常的原理和【方式二:运行时异常】一样
1 |
|
其他异常
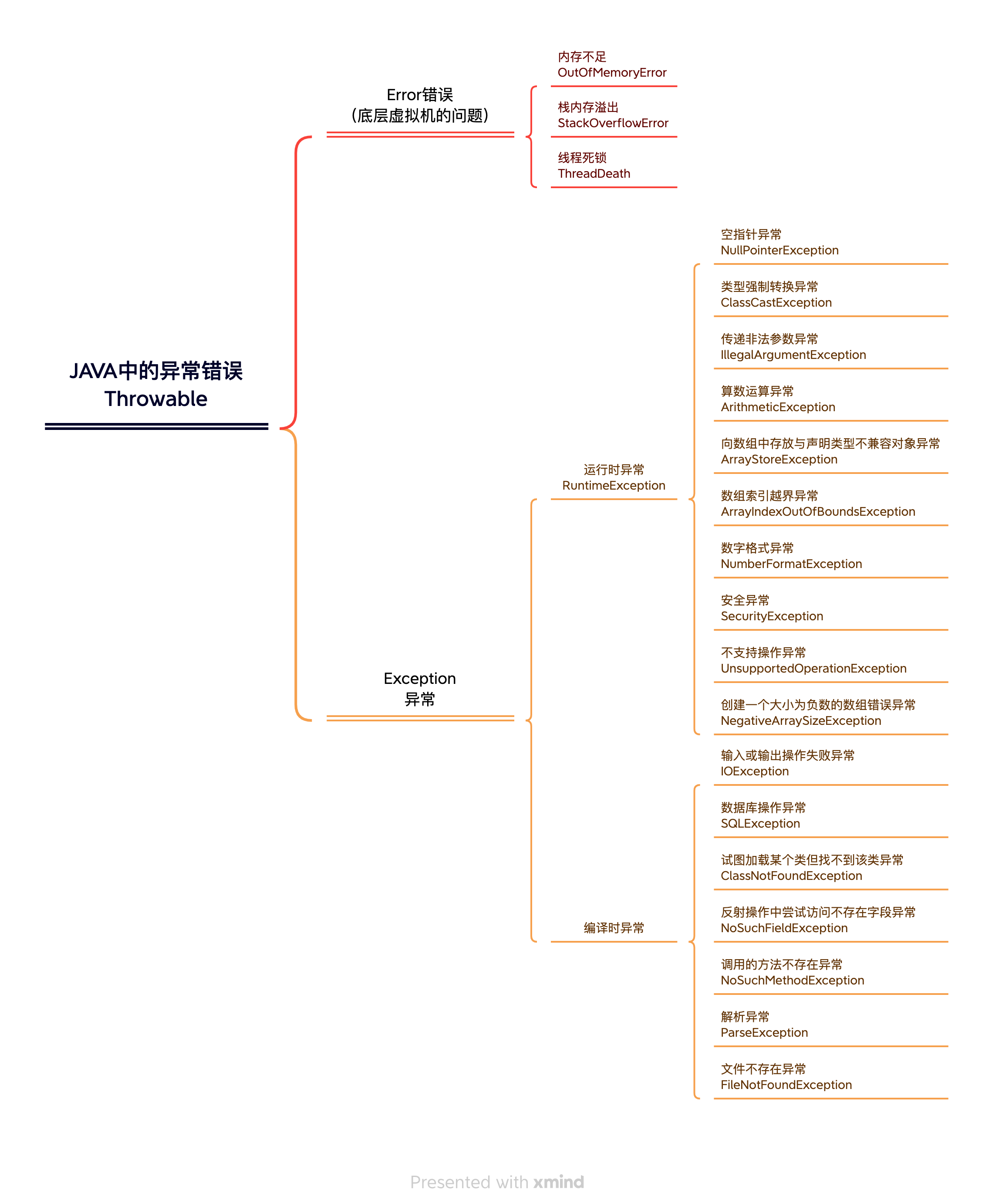
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 学弟不想努力了!
评论