Java中读存properties文件的配置信息
前言
application.properties 文件位于何处?
properties 是一种语法格式,用于以平面.properties存储属性。
属性文件位于我们应用程序的src/main/resources
文件夹中。
该文件允许我们配置 Spring 并为我们的应用程序定义我们自己的配置。
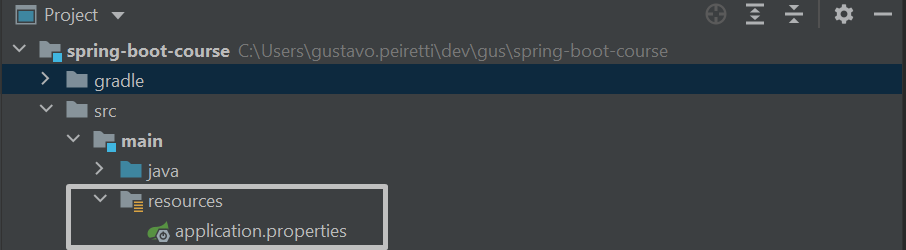
有大量的属性可以设置,我们可以在这里看到一个列表。请注意,有 16 个类别对属性进行分类,从 Sprint 核心到数据配置再到测试。通过数据配置到测试。
我们的属性文件的示例可能如下所示:
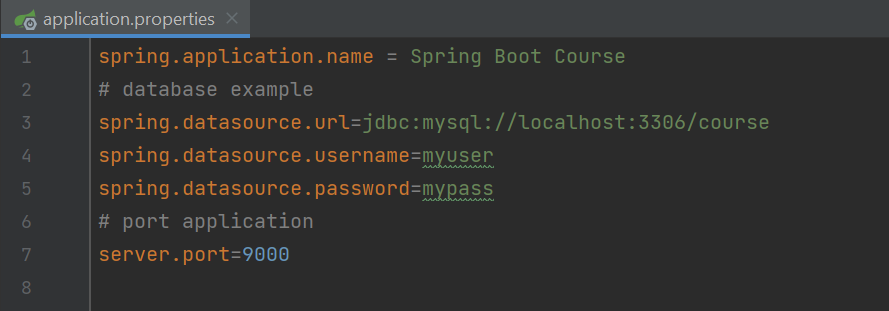
在这个application.properties文件中,我们使用 Spring 已经定义的属性为我们的应用程序命名,更改它运行的端口并为其提供数据库设置。为我们的应用程序命名,更改其运行的端口并指示数据库配置。
配置结构
配置文件:config.properties
配置文件的路径:src/main/resources/static/config.properties
配置文件config.properties
内容如下:(用来测试的需要读取的数据,key-value
):
读取properties文件中的某个值
config.properties配置1
ip = 127.0.0.1
方法一
引入包1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.Properties;
/**
* 读取config.properties文件中ip值
*/
public void readProperties() {
Properties pro = new Properties();
// 使用InPutStream流读取properties文件
BufferedReader bufferedReader;
try {
//读取本地目录下的properties文件
bufferedReader = new BufferedReader(new FileReader("D:/config.properties"));
//读取项目中resources下的properties文件
//bufferedReader = new BufferedReader(new FileReader("src/main/resources/config.properties"));
pro.load(bufferedReader);
} catch (IOException e) {
e.printStackTrace();
}
String ip = String.valueOf(pro.get("ip"));
}
方法二:
1 | /** |
修改properties文件中的某个值
config.properties配置1
ip = 127.0.0.1
方法一
1 | //引入包 |
方法二
1 | /** |
使用阿里的fastjson解析
config.properties配置1
2
3
4
5ip = 127.0.0.1
port = 8080
filepath = D:/download
username = root
password = 1234561
2
3
4
5
6<!-- fastjson包 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.83</version>
</dependency>1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.Properties;
import com.alibaba.fastjson.JSONObject;
public class getPropertys {
public static void main(String[] args) throws IOException {
Properties pro = new Properties();
// 使用InPutStream流读取properties文件
BufferedReader bufferedReader = new BufferedReader(new FileReader("src/main/resources/static/config.properties"));
pro.load(bufferedReader);
JSONObject obj = new JSONObject();
//取出properties中的数据,并赋值
obj.put("ip",pro.getProperty("ip"));
obj.put("port",pro.getProperty("port"));
obj.put("filepath",pro.getProperty("filepath"));
obj.put("username",pro.getProperty("username"));
obj.put("password",pro.getProperty("password"));
System.out.println("输出Object对象:" + obj.toString());
}
}
使用Google的gson解析
config.properties配置1
2
3
4
5ip = 127.0.0.1
port = 8080
filepath = D:/download
username = root
password = 1234561
2
3
4
5
6<!-- gson包 -->
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.5</version>
</dependency>1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.Properties;
import com.google.gson.JsonObject;
public class getPropertys {
public static void main(String[] args) throws IOException {
Properties properties = new Properties();
// 使用InPutStream流读取properties文件
BufferedReader bufferedReader = new BufferedReader(new FileReader("src/main/resources/static/config.properties"));
properties.load(bufferedReader);
JSONObject obj = new JSONObject();
JsonObject obj=new JsonObject();
obj.addProperty("ip",properties.getProperty("ip"));
obj.addProperty("port",properties.getProperty("port"));
obj.addProperty("filepath",properties.getProperty("filepath"));
obj.addProperty("username",properties.getProperty("username"));
obj.addProperty("password",properties.getProperty("password"));
System.out.println("输出Object对象:" + obj.toString());
}
}