Java中读取yml文件的配置信息
前言
YAML 是一种语法格式,与properties文件
大致一样,用于以分层格式存储属性。application.yml
大致结构如下所示:
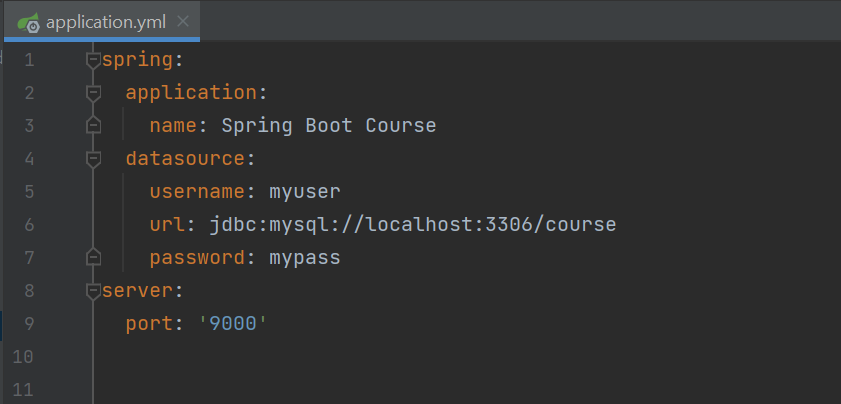
配置结构
配置文件:application.yml
配置文件的路径:src/main/resources/application.yml
配置文件application.yml
内容如下:(用来测试的需要读取的数据,树形结构key-value
):
例子:1
2
3
4
5
6
7
8
9
10
11
12smart:
ip: 127.0.0.1
port: 8080
size: [1,3,4,5,8]
url:
index: http://blog.renyuxin.cn
image: http://blog.renyuxin.cn/image
users:
- id: 1
name: 超级管理员
- id: 2
name: 测试员
方式一:@Value 注解
通过@Value
注解的${key}
即可获取application.yml配置文件
中和key
对应的value
值
注意:这个方法适用于微服务比较少的情形.1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
public class ConfigProperties {
private String ip;
private String port;
private String indexUrl;
private String size;
private String adminId;
private String admin;
public void getConfigProperties(){
System.out.println(ip);
System.out.println(port);
System.out.println(indexUrl);
System.out.println(size);
System.out.println(adminId);
System.out.println(admin);
}
}
调用1
2
3
4
5
6
7
8
9
10
11import com.smart.Demo.util.DemoUtils;
import org.springframework.beans.factory.annotation.Autowired;
public class DemoService {
ConfigProperties config;
public void getDemo(){
config.getDemo();
}
}
输出结果:
127.0.0.1
8080
http://blog.renyuxin.cn
4
1
1 - 超级管理员
方式二:使用 Environment对象
1 |
|
使用Environment对象的getProperty(String key)
通过key获取值1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
public class DemoService {
private Environment env;
public void getConfig(){
System.out.println(env.getProperty("smart.ip"));
System.out.println(env.getProperty("smart.port"));
System.out.println(env.getProperty("smart.url.index"));
System.out.println(env.getProperty("smart.size[2]"));
System.out.println(env.getProperty("smart.users[0].id"));
}
}
方式三:实体类封装 @Component + @ConfigurationProperties
封装实体
使用@Component
注解表示该对象为spring
的Bean
使用@ConfigurationProperties
注解绑定配置信息到封装类中
@Component
可替换成 @Configuration
、@Service
等注解
1 | import lombok.Data; |
调用
1 | import com.smart.Demo.domian.Demo; |
输出
Demo(ip=127.0.0.1, port=8080, url={index=http://blog.renyuxin.cn, image=http://blog.renyuxin.cn/image}, users=[{id=1, name=超级管理员}, {id=2, name=测试员}])
方式四:Application启动类 @EnableConfigurationProperties
Application
直接扫描类
或者扫描类所在的路径
1 |
|
1 |
|